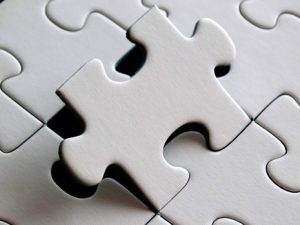
This is a small tutorial regarding “components” property within a View Page for new users. In previous tutorial, you’ll notice the typical boilerplate code for ViewPage (see below – line 10) contains the “components” property.
var kind = require('enyo/kind'); var Button = require('enyo/button'); var FittableRows = require('layout/FittableRows'); module.exports = kind({ name: 'FirstPage', data: null, kind: FittableRows, classes: 'enyo-fit', components:[ { // You may add your own inline css styling like this below. style:"background:#f2f2f2;text-align:center;height:40px;line-height:40px", content:"Hello World" }, { // fit: true is used to enyo to auto fill all the remaining height of the page. fit:true, style:"background:#cccccc;text-align:center;padding:10px;", components:[ { kind:Button, content:"Click Me", ontap:"handle_ClickMe" } ] } ], create: function(){ this.inherited(arguments); }, rendered : function(){ this.inherited(arguments); }, handle_ClickMe:function(inSender,inEvent){ console.log("This is how button events are done"); } });
During ViewPage runtime, Enyo will take all the controls declared within the components property and render into the document’s body. Simplest way of visualizing a components property is to picture it as a huge DIV tag.
What goes into components ?
Let’s modify the code and see the results. Delete the whole components property line 10 – 28. Insert code below. This will just render a div tag that contains the text “This is a text within a div tag”.
components:[ { content: "This is a text within a div tag" } ]
Render content attributes with html tags
You can also render elements within “content:” See sample below.
components:[ { content: "<p>This is a paragraph text portion is <strong>BOLD</strong> and <u>underlined</u></p>", allowHtml:true } ]
Nested Components
Let’s move on to next code… How about we want our viewpage to render some html codes that looks like this…
<div class="center-div"> <h1 class="big-header red-text">This is a header</h1> </div>
To achieve what’s written above, your components structure should look like…Notice you can wrap components property with another just like how we wrap our div around the header.
components:[ { classes: "center-div", components:[ { tag: "h1", classes: "big-header red-text", content:"This is a header" } ] } ]
Extra HTML Attributes
Sometimes, some tags have more additional html attributes other than just textual content and css classes. For example for situation like this …
<p>This is an example p tag above an image…</p> <img src="http://www.somewhere.com/images/header.png" width="360" height="240" />
For this we use attributes properties.
components:[ { tag: "p", content:"This is an example p tag above an image…" }, { tag: "img", attributes:[ { src:"http://www.somewhere.com/images/header.png", width:"360", height:"240" } ] } ]
Using Enyo Libraries
You can mix enyo control classes like enyo.Button, enyo.Input together with tag to craft your view page content too.
// If you want to use enyo.Input for example, don't forget to include it into your ViewPage for it to work. var Input = require('enyo/Input');
And the components region should look like this…
components: [ { kind:Input, name:"txtInput", classes:"fixed-width", placeholder: "Insert value", value:"", type: "text", attributes:{ maxlength:256, /* you can insert non-w3c standard attributes too since is usable in html5 for scripting usage */ data-required: "none" } } ]
When to know to place property inside attributes and when to place outside?
Technically, any non-supported property of the kind can be listed within attributes. Take a look at enyo.Input above. It has default property for name, classes, placeholder, value, type and disabled, events to be accessed programmatically. Anything out of the scope can still be inserted using attributes.